object::RingBuffer< T, N > Class Template Reference
Ring buffer (constant size). More...
#include <RingBuffer.h>
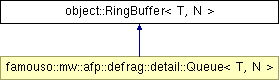
Public Types | |
typedef SmallestUnsignedTypeSelector < N >::type | SizeT |
Type used for internal indexing. | |
Public Member Functions | |
RingBuffer () | |
Constructor. | |
SizeT | get_count () const |
Returns current count of elements. | |
bool | is_empty () const |
Returns true if there are no elements inside. | |
bool | is_full () const |
Returns true if no more elements can be added. | |
T & | front () |
Returns reference to the first element. | |
const T & | front () const |
Returns reference to the first element. | |
T & | back () |
Returns reference to the last element. | |
const T & | back () const |
Returns reference to the last element. | |
T & | operator[] (SizeT i) |
Returns reference to element i . | |
const T & | operator[] (SizeT i) const |
Returns reference to element i . | |
void | push_front (const T &obj) |
Adds an element in front of the first one. | |
void | push_back (const T &obj) |
Adds an element behind the last one. | |
void | pop_front () |
Removes the first element. | |
void | pop_back () |
Removes the last element. | |
Protected Types | |
typedef ExpandedRangeTypeSelector < SizeT >::type | DoubleSizeT |
Type with larger range than SizeT to handle overflows. | |
Protected Member Functions | |
SizeT | get_idx (SizeT num) const |
Get index of element. | |
void | increment_first () |
Increments first . | |
void | decrement_first () |
Decrements first . | |
SizeT | get_last () const |
Return index of last element. | |
SizeT | get_behind_last () const |
Return index of element behind last element. | |
SizeT | get_infront_first () const |
Return index of element in front of first element. | |
Protected Attributes | |
T | buffer [N] |
Array containing elements | |
SizeT | first |
Array index of first element (always < N) | |
SizeT | count |
Number of elements currently used (always <= N). |
Detailed Description
template<class T, unsigned int N>
class object::RingBuffer< T, N >
Ring buffer (constant size).
- Template Parameters:
-
T Type of elements N Maximum count of elements
Member Typedef Documentation
typedef ExpandedRangeTypeSelector<SizeT>::type object::RingBuffer< T, N >::DoubleSizeT [protected] |
Type with larger range than SizeT to handle overflows.
typedef SmallestUnsignedTypeSelector<N>::type object::RingBuffer< T, N >::SizeT |
Type used for internal indexing.
Constructor & Destructor Documentation
object::RingBuffer< T, N >::RingBuffer | ( | ) | [inline] |
Constructor.
Member Function Documentation
const T& object::RingBuffer< T, N >::back | ( | ) | const [inline] |
Returns reference to the last element.
- Precondition:
- is_empty() returns false
T& object::RingBuffer< T, N >::back | ( | ) | [inline] |
Returns reference to the last element.
- Precondition:
- is_empty() returns false
void object::RingBuffer< T, N >::decrement_first | ( | ) | [inline, protected] |
Decrements first
.
Referenced by object::RingBuffer< Event< KeyType > *, N >::push_front().
const T& object::RingBuffer< T, N >::front | ( | ) | const [inline] |
Returns reference to the first element.
- Precondition:
- is_empty() returns false
T& object::RingBuffer< T, N >::front | ( | ) | [inline] |
Returns reference to the first element.
- Precondition:
- is_empty() returns false
SizeT object::RingBuffer< T, N >::get_behind_last | ( | ) | const [inline, protected] |
Return index of element behind last element.
Referenced by object::RingBuffer< Event< KeyType > *, N >::push_back().
SizeT object::RingBuffer< T, N >::get_count | ( | ) | const [inline] |
Returns current count of elements.
SizeT object::RingBuffer< T, N >::get_idx | ( | SizeT | num | ) | const [inline, protected] |
Get index of element.
- Parameters:
-
num Number of element behind first (first = 0, last = count-1) Must be smaller than N
.
- Returns:
- Index of element in
buffer
Referenced by object::RingBuffer< Event< KeyType > *, N >::get_behind_last(), object::RingBuffer< Event< KeyType > *, N >::get_infront_first(), object::RingBuffer< Event< KeyType > *, N >::get_last(), and object::RingBuffer< Event< KeyType > *, N >::operator[]().
SizeT object::RingBuffer< T, N >::get_infront_first | ( | ) | const [inline, protected] |
Return index of element in front of first element.
Referenced by object::RingBuffer< Event< KeyType > *, N >::push_front().
SizeT object::RingBuffer< T, N >::get_last | ( | ) | const [inline, protected] |
Return index of last element.
Referenced by object::RingBuffer< Event< KeyType > *, N >::back().
void object::RingBuffer< T, N >::increment_first | ( | ) | [inline, protected] |
Increments first
.
Referenced by object::RingBuffer< Event< KeyType > *, N >::pop_front().
bool object::RingBuffer< T, N >::is_empty | ( | ) | const [inline] |
Returns true if there are no elements inside.
Referenced by famouso::mw::afp::defrag::detail::Queue< Event< KeyType > *, DCP::old_event_ids >::empty(), object::RingBuffer< Event< KeyType > *, N >::front(), object::RingBuffer< Event< KeyType > *, N >::get_last(), object::RingBuffer< Event< KeyType > *, N >::pop_back(), and object::RingBuffer< Event< KeyType > *, N >::pop_front().
bool object::RingBuffer< T, N >::is_full | ( | ) | const [inline] |
Returns true if no more elements can be added.
Referenced by famouso::mw::afp::defrag::detail::Queue< Event< KeyType > *, DCP::old_event_ids >::full(), object::RingBuffer< Event< KeyType > *, N >::push_back(), and object::RingBuffer< Event< KeyType > *, N >::push_front().
const T& object::RingBuffer< T, N >::operator[] | ( | SizeT | i | ) | const [inline] |
Returns reference to element i
.
- Parameters:
-
i Index of element. Zero for the first element, count-1
for the last.
T& object::RingBuffer< T, N >::operator[] | ( | SizeT | i | ) | [inline] |
Returns reference to element i
.
- Parameters:
-
i Index of element. Zero for the first element, count-1
for the last.
void object::RingBuffer< T, N >::pop_back | ( | ) | [inline] |
Removes the last element.
- Precondition:
- is_empty() returns false
void object::RingBuffer< T, N >::pop_front | ( | ) | [inline] |
Removes the first element.
- Precondition:
- is_empty() returns false
Referenced by famouso::mw::afp::defrag::detail::Queue< T, dynamic >::pop(), and famouso::mw::afp::defrag::detail::Queue< Event< KeyType > *, DCP::old_event_ids >::pop().
void object::RingBuffer< T, N >::push_back | ( | const T & | obj | ) | [inline] |
Adds an element behind the last one.
- Parameters:
-
obj Object to insert
- Precondition:
- is_full() returns false
Referenced by famouso::mw::afp::defrag::detail::Queue< T, dynamic >::push(), and famouso::mw::afp::defrag::detail::Queue< Event< KeyType > *, DCP::old_event_ids >::push().
void object::RingBuffer< T, N >::push_front | ( | const T & | obj | ) | [inline] |
Adds an element in front of the first one.
- Parameters:
-
obj Object to insert
- Precondition:
- is_full() returns false
Field Documentation
T object::RingBuffer< T, N >::buffer[N] [protected] |
Array containing elements
Referenced by object::RingBuffer< Event< KeyType > *, N >::back(), object::RingBuffer< Event< KeyType > *, N >::front(), object::RingBuffer< Event< KeyType > *, N >::operator[](), object::RingBuffer< Event< KeyType > *, N >::push_back(), and object::RingBuffer< Event< KeyType > *, N >::push_front().
SizeT object::RingBuffer< T, N >::count [protected] |
Number of elements currently used (always <= N).
Referenced by object::RingBuffer< Event< KeyType > *, N >::get_behind_last(), object::RingBuffer< Event< KeyType > *, N >::get_count(), object::RingBuffer< Event< KeyType > *, N >::get_last(), object::RingBuffer< Event< KeyType > *, N >::is_empty(), object::RingBuffer< Event< KeyType > *, N >::is_full(), object::RingBuffer< Event< KeyType > *, N >::pop_back(), object::RingBuffer< Event< KeyType > *, N >::pop_front(), object::RingBuffer< Event< KeyType > *, N >::push_back(), and object::RingBuffer< Event< KeyType > *, N >::push_front().
SizeT object::RingBuffer< T, N >::first [protected] |
Array index of first element (always < N)
Referenced by object::RingBuffer< Event< KeyType > *, N >::decrement_first(), object::RingBuffer< Event< KeyType > *, N >::front(), object::RingBuffer< Event< KeyType > *, N >::get_idx(), and object::RingBuffer< Event< KeyType > *, N >::increment_first().
The documentation for this class was generated from the following file:
- include/object/RingBuffer.h