famouso::mw::attributes::access::Attribute_RT Class Reference
A structure that extends the AttributeHeader_RT type providing access to the encoded value of the attribute. More...
#include <Attribute_RT.h>
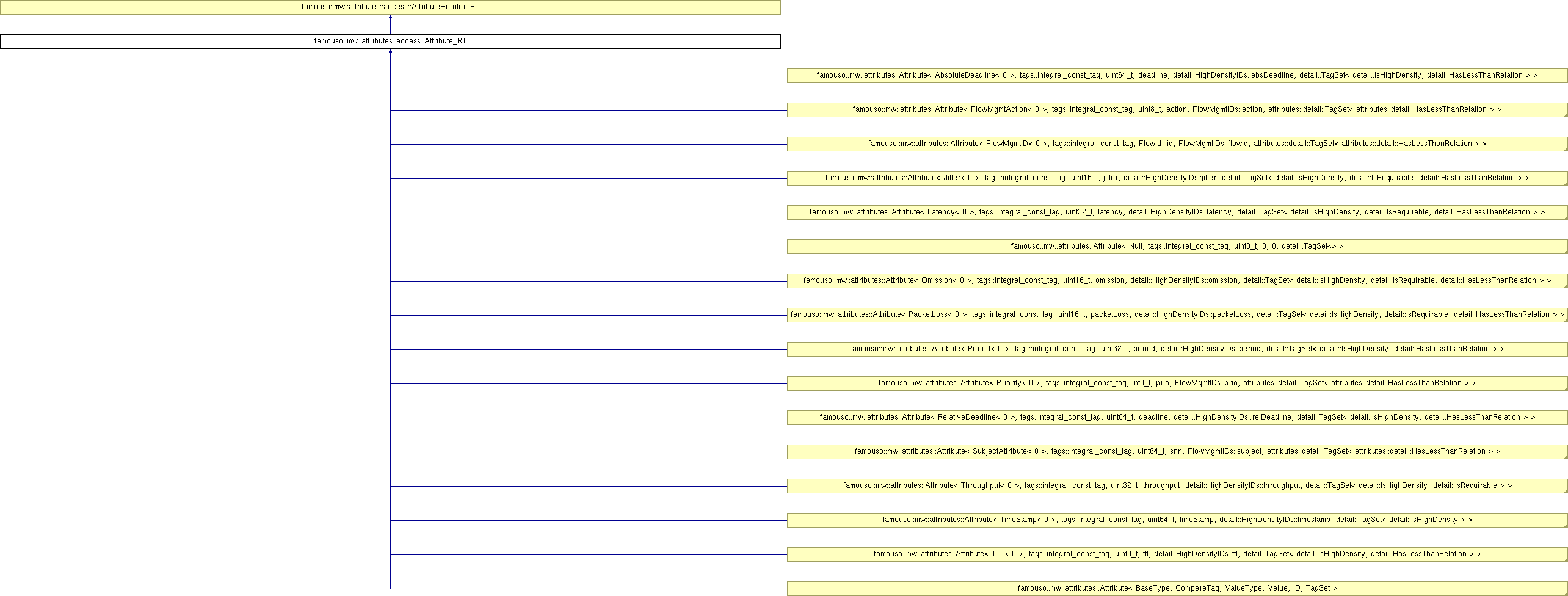
Public Member Functions | |
template<typename ValueType > | |
const ValueType | getValue () const |
Returns the value from the binary representation of this attribute instance. | |
template<typename ValueType > | |
const bool | setValue (const ValueType newValue) |
Sets the value in the binary representation of this attribute to the given one. | |
uint16_t | length () const |
Determines the number of bytes used by the binary representation of this attribute. | |
Protected Member Functions | |
Attribute_RT () |
Detailed Description
A structure that extends the AttributeHeader_RT type providing access to the encoded value of the attribute.
As the AttributeHeader_RT type this type is also not constructible. Since AttributeHeader_RT is extended by this type without adding actual data deriving types must also care that 'this' points to an encoded attribute.
- Todo:
- The implementation of getValue and setValue should be more resource efficient because if different Attributes are in use, the methods are compiler-generated multiple times. However, this needs not to be so. A possible solution would be to factor out the common parts and only the for type-safety let the compiler generate a small cast method.
Constructor & Destructor Documentation
famouso::mw::attributes::access::Attribute_RT::Attribute_RT | ( | ) | [inline, protected] |
Member Function Documentation
const ValueType famouso::mw::attributes::access::Attribute_RT::getValue | ( | ) | const [inline] |
Returns the value from the binary representation of this attribute instance.
The value is parsed from the binary representation of this attribute. That means this attribute's static properties are not used at all.
- Template Parameters:
-
ValueType The type of this attribute's value
- Returns:
- This attribute's value
Reimplemented in famouso::mw::attributes::Attribute< BaseType, CompareTag, ValueType, Value, ID, TagSet >, famouso::mw::attributes::Attribute< PacketLoss< 0 >, tags::integral_const_tag, uint16_t, packetLoss, detail::HighDensityIDs::packetLoss, detail::TagSet< detail::IsHighDensity, detail::IsRequirable, detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< Period< 0 >, tags::integral_const_tag, uint32_t, period, detail::HighDensityIDs::period, detail::TagSet< detail::IsHighDensity, detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< FlowMgmtID< 0 >, tags::integral_const_tag, FlowId, id, FlowMgmtIDs::flowId, attributes::detail::TagSet< attributes::detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< Jitter< 0 >, tags::integral_const_tag, uint16_t, jitter, detail::HighDensityIDs::jitter, detail::TagSet< detail::IsHighDensity, detail::IsRequirable, detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< TTL< 0 >, tags::integral_const_tag, uint8_t, ttl, detail::HighDensityIDs::ttl, detail::TagSet< detail::IsHighDensity, detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< TimeStamp< 0 >, tags::integral_const_tag, uint64_t, timeStamp, detail::HighDensityIDs::timestamp, detail::TagSet< detail::IsHighDensity > >, famouso::mw::attributes::Attribute< Priority< 0 >, tags::integral_const_tag, int8_t, prio, FlowMgmtIDs::prio, attributes::detail::TagSet< attributes::detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< FlowMgmtAction< 0 >, tags::integral_const_tag, uint8_t, action, FlowMgmtIDs::action, attributes::detail::TagSet< attributes::detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< Null, tags::integral_const_tag, uint8_t, 0, 0, detail::TagSet<> >, famouso::mw::attributes::Attribute< Throughput< 0 >, tags::integral_const_tag, uint32_t, throughput, detail::HighDensityIDs::throughput, detail::TagSet< detail::IsHighDensity, detail::IsRequirable > >, famouso::mw::attributes::Attribute< Latency< 0 >, tags::integral_const_tag, uint32_t, latency, detail::HighDensityIDs::latency, detail::TagSet< detail::IsHighDensity, detail::IsRequirable, detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< RelativeDeadline< 0 >, tags::integral_const_tag, uint64_t, deadline, detail::HighDensityIDs::relDeadline, detail::TagSet< detail::IsHighDensity, detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< Omission< 0 >, tags::integral_const_tag, uint16_t, omission, detail::HighDensityIDs::omission, detail::TagSet< detail::IsHighDensity, detail::IsRequirable, detail::HasLessThanRelation > >, famouso::mw::attributes::Attribute< SubjectAttribute< 0 >, tags::integral_const_tag, uint64_t, snn, FlowMgmtIDs::subject, attributes::detail::TagSet< attributes::detail::HasLessThanRelation > >, and famouso::mw::attributes::Attribute< AbsoluteDeadline< 0 >, tags::integral_const_tag, uint64_t, deadline, detail::HighDensityIDs::absDeadline, detail::TagSet< detail::IsHighDensity, detail::HasLessThanRelation > >.
References famouso::mw::attributes::access::AttributeHeader_RT::asElementHeader(), famouso::mw::attributes::detail::AttributeElementHeader::extension, famouso::mw::attributes::detail::AttributeElementHeader::isHighDensity(), length(), and famouso::mw::attributes::detail::AttributeElementHeader::lengthValueSwitch.
uint16_t famouso::mw::attributes::access::Attribute_RT::length | ( | ) | const [inline] |
Determines the number of bytes used by the binary representation of this attribute.
- Returns:
- This attribute's size in bytes
References famouso::mw::attributes::access::AttributeHeader_RT::headerLength(), and famouso::mw::attributes::access::AttributeHeader_RT::valueLength().
Referenced by getValue(), and setValue().
const bool famouso::mw::attributes::access::Attribute_RT::setValue | ( | const ValueType | newValue | ) | [inline] |
Sets the value in the binary representation of this attribute to the given one.
Since the binary representation of attribute's value has some restrictions in size it could be the case that it is not possible to apply the given value. This could for example be the case if the current value is written unextended and the new value would demand to be written extended.
- Template Parameters:
-
ValueType The type of this attribute's value
- Parameters:
-
newValue The new value to set
- Returns:
- True if the value could properly be set, false if the value could not be set and the attribute keeps its previous value
Reimplemented in famouso::mw::attributes::Attribute< BaseType, CompareTag, ValueType, Value, ID, TagSet >.
References famouso::mw::attributes::access::AttributeHeader_RT::asElementHeader(), bitCountToByteCount(), famouso::mw::attributes::detail::AttributeElementHeader::extension, getBitCount(), famouso::mw::attributes::detail::AttributeElementHeader::isHighDensity(), length(), famouso::mw::attributes::detail::AttributeElementHeader::lengthValueSwitch, and famouso::mw::attributes::detail::AttributeElementHeader::valueOrLength.
The documentation for this class was generated from the following file:
- include/mw/attributes/access/Attribute_RT.h