AFP configuration
How to configure AFP
AFP has an template-based configuration mechanism. Thus the configuration is known at compile time which allows to build an optimized version of AFP based on the configuration options you choose.
An AFP configuration is a C++ struct type that defines at least a fixed set of enum values and may contain several policy class definitions. It is recommended to use one of the predefined config types if they fit you requirements. Otherwise you can create your own config by defining a new struct that is derived from an existing config type and overwriting certain configuration options.
The predefined configurations are listed in section Predefined AFP configs. Here is an example of a user-defined AFP configuration:
// Define "MyAFPConfig", use DefaultConfig as base struct MyAFPConfig : famouso::mw::afp::DefaultConfig { // Overwrite several boolean config options enum { event_seq = true, duplicates = true, reordering = true }; // Type option: use own allocator typedef MyAllocator Allocator; };
Most configuration options are enumeration values (Boolean options and Unsigned integer options for tuning), but some are types (Type options).
Configuration options
This section explains the configuration options you have.
Boolean options
event_seq
If the event_seq
option is set to true
, fragmentation will use event sequence numbering and defragmentation will support both fragments with and without event sequence number extension header. It also enables support for concurrent reassembly of multiple events. If it is false
, fragments will not contain an event sequence number extension header and the defragmentation will drop fragments containing such a header.
Event sequence numbers are needed for event identification. The receiver has to assign each fragment to an event if multiple events are reassembled concurrently.
Setting event_seq = true
is recommended in the following situations:
- The network suffers from packet loss and you want to send events in a high rate. Without
event_seq = true
AFP cannot ensure that reassembled events are correct.
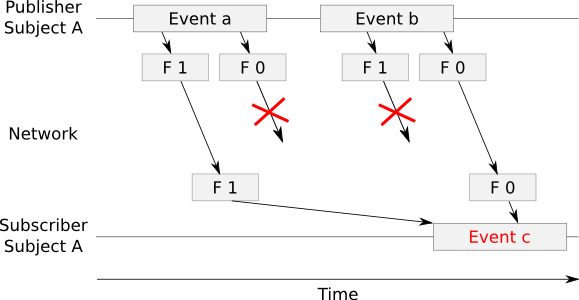
Example packet loss scenario which needs event sequence numbering
- The network suffers from packet reordering and you want to send events in a high rate. If only one event is involved reordering can be handled by without event sequence numbers. Otherwise they are needed to ensure correct reassembly.
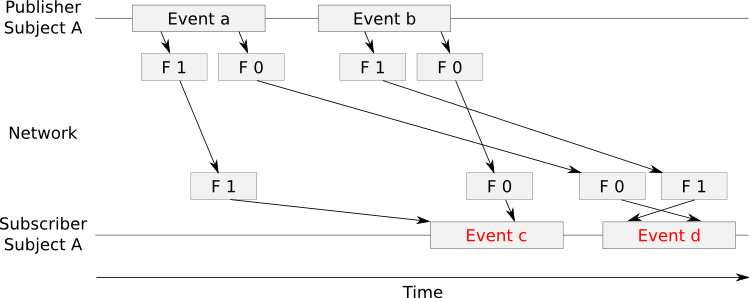
Example packet reordering scenario which needs event sequence numbering
- Multiple events with the same subject are fragmented concurrently and fragments are sent interleaved from one network node. If fragmentation takes place in Application Layer, all publishers on one subject must be part of one application (process) and use the same AFP configuration to make them share the event sequence number counter. If fragmentation is only done in the Abstract Network Layer, multiple publishers from different applications may publish events concurrently. That is because in the current implementation multiple events are not fragmented concurrently in the Abstract Network Layer, due to the fact that the ECHs serializes the processing of publish commands. Thus, fragments are never transmitted interleaved from the Abstract Network Layer. But cause this could change in future versions, it is recommended to use event sequence numbering in such cases as well.
Fragmentation for multiple publishers from different network nodes on one subject is not not covered by the event sequence numbering extension header and thus not supported at the moment. It can result in the delivery of corrupted events to subscribers.
Although this option is called event sequence numbering, it does not guarantee any order. The sequence of event delivery to subscribers can differ from the sequence of event publishing.
If the event_seq
option is set to true
, you may consider to change to options concurrent_events
and old_event_ids
for fine tuning.
For some more information about AFP's event sequence number extension, see Event Sequence Numbers.
reordering
This option determines whether the defragmentation supports handling reordered packages. If reordering
is set to true
, the event reassembly policy is able to order the fragments. In case this option is false
AFP assumes that network packet order is retained. Thus, if the order of fragment sequence numbers differs from the expectations, AFP assumes packet loss to be the reason and drops the incomplete event immediately.
Enabling this option does not ensure correct reassembly of events if they are published in a high rate, because it only handles reordering of fragments belonging to one fragment. See event_seq for details about this issue.
duplicates
Use duplicates = true
if the same packets may be delivered to AFP multiple time. It enables checking for duplicate fragments in the event reassembly. It does not ensure that events which are published only one time are delivered only once to the subscribers. For example, if duplicates of all fragments of an event arrive after the events first delivery, event_seq must be employed to avoid duplicate delivery. For resource constrained platforms see max_fragments.
This option does not provide any duplicate checking for famouso events that are not fragmented via AFP.
FEC
Setting FEC
to true
enables the fragmentation to create forward error correction redundancy fragments. Use it to increase the probability not to loose an event in a lossy network channel at the expense of higher network traffic. At the receiver side enabling this option is necessary to reassemble events transmitted with redundancy fragments, even if the redundancy is not needed because no message fragment was lost. FEC = true
implies reordering = true
.
To set the percentage of redundancy fragments to create by the AFP fragmentation, you have to access the redundancy attribute associated with the used fragmenter. If it is not given explicitly in the AFP config (RedundancyAttribute), the default attribute is used (famouso::mw::afp::FragmentationRedundancy). Initially it is set to zero percent, resulting in no redundancy fragments and the omission of the forward error correction extension header. You can change the value of the default redundancy attribute like this:
famouso::mw::afp::FragmentationRedundancy::value() = 20; // 20 percent redundancy
The value must be in range of 0 to 255.
For some more information about AFP's forward error correction extension, see Forward Error Correction.
multiple_subjects
The option multiple_subjects
defines whether the defragmentation part has to distinguish different fragment sources. The famouso Abstract Network Layer needs this feature if multiple subjects are used. If it is enabled, the SubjectType has to be given, because it is used to distinguish the sources.
defrag_statistics
The option defrag_statistics
defines if the defragmentation part will collect statistics about the arisen fragments and reassembly of events. See DefragStatistics for using multiple separated statistics. By default famouso::mw::afp::DefragmentationStatistics is used to collect data.
Example how to access the statistics:
using namespace famouso::mw; // Get current fragment statistics afp::defrag::FragmentStats fs; afp::DefragmentationStatistics::get_fragment_stats(fs); // Get current event statistics afp::defrag::EventStats es; afp::DefragmentationStatistics::get_event_stats(es);
See also famouso::mw::afp::defrag::FragmentStats and famouso::mw::afp::defrag::EventStats for more information about the collected statistics.
overflow_error_checking
This option can be set to false
to disable overflow error checking during several calculations that are needed for fragmentation. By default AFP checks, whether the data types given by the SizeProperties are sufficient in range for the needed calculations. Disabling is only recommended if you tested the maximum event size that needs to be fragmented and no error occurred. Do not change configuration options after disabling overflow error checking!
Unsigned integer options for tuning
concurrent_events
This option defines the maximum number of events that can be reassembled by the AFP defragmentation concurrently. It is only used if either event_seq or multiple_subjects is set to true
. Otherwise only one event can be reassembled at a time and this option is ignored.
Next to unsigned integer values which define a constant maximum count of events, you can also assign the constant famouso::mw::afp::dynamic
to this option. In this case a data structure with dynamic size is used, that requires memory allocations.
old_event_ids
This option can be used to configure the maximum count of event identifications (event sequence numbers) that are locked, which means they were recently used by events that got reassembled successfully or dropped because of timeout. This option is ignored if event_seq is not set to true
.
The locking is used to prevent that late fragments, duplicates or unneeded redundancy fragments lead to creating a new event defragmenter.
Next to unsigned integer values which define a constant maximum count of events, you can also assign the constant famouso::mw::afp::dynamic
to this option. In this case a data structure with dynamic size is used, that requires memory allocations.
max_fragments
This option defines the maximum number of fragments that an event can consist of. It is only used by the fragment duplicate checking and thus ignored if duplicates is not set to true
.
Next to unsigned integer values which define a constant maximum count of events, you can also assign the constant famouso::mw::afp::dynamic
to this option. In this case a data structure with dynamic size is used, that requires memory allocations.
Type options
SizeProperties
AFP allows to define the data types to use for event sizes, fragment sizes and fragment counts. This firstly influences the range of possible values for these quantities. You can choose larger data types to enable fragmentation of larger events in the Application Layer. Secondly it also influences the RAM usage and code size which is mainly interesting for resource constrained embedded systems.
The data types can be configured by a struct type that defines the types elen_t
(the event length type), flen_t
(the fragment length type and fcount_t
(the fragment count type). Such a struct is called a size properties struct. Some of these structs are predefined:
- famouso::mw::afp::DefaultEventSizeProp
If no size properties config is given explicitly, this is used. It matches the definition of famouso's maximum event length. - famouso::mw::afp::MinimalSizeProp
All three types are unsigned 8-bit integer here. This is suitable for 8-bit micro-controllers, in case events are smaller than 256 bytes. - famouso::mw::afp::SizeProp
Selects the data types from a given maximum event size and the MTU.
To change the size properties configuration used by AFP, define a size properties struct with the identifier SizeProperties
in the AFP config. This typedef option is used by both, the fragmentation and the defragmentation.
Example:
struct AFPConfig : afp::DefaultConfig { typedef afp::MinimalSizeProp SizeProperties; };
Allocator
The type option Allocator
allows to overwrite the default allocator type that is utilized by AFP (in the defragmentation and the AFPPublisherEventChannel version with run-time MTU selection).
Some allocator types are defined in the header file include/object/Allocator.h
. On the AVR platform object::RawStorageAllocator is the default Allocator. For all other platforms object::NewAllocator is employed by default. The default RawStorageAllocator memory pool size is 255 bytes on AVR and 65535 bytes on other platforms. It can be overwritten by defining DEFAULT_RAWSTORAGEALLOCATOR_MEM_SIZE before including Allocator.h
.
Example:
struct AFPConfig : afp::DefaultConfig { typedef object::OneBlockAllocator<afp::EmptyType, EVENT_LENGTH> Allocator; };
RedundancyAttribute
If FEC
is set to true
you can define an own redundancy attribute for fragmentation, e.g. if you use multiple AFP configurations with different forward error correction redundancy levels. It allows to set different initial redundancy percentage and to change the values independently.
Example:
struct MyAFPConfig1 : afp::DefaultConfig { enum { event_seq = true, FEC = true }; typedef famouso::mw::afp::frag::RedundancyAttribute<MyAFPConfig1, 10> RedundancyAttribute; }; struct MyAFPConfig2 : afp::DefaultConfig { enum { event_seq = true, FEC = true }; typedef famouso::mw::afp::frag::RedundancyAttribute<MyAFPConfig2, 30> RedundancyAttribute; }; // ... MyAFPConfig2::RedundancyAttribute::value() = 10; // Change redundancy percentage
SubjectType
For defragmentation with support for multiple fragment sources (multiple_subjects
) you have to define a type that can be used to distinguish the different fragment sources. AFP assumes to find this type inside the AFP config with the identifier SubjectType
.
Example:
template < typename SNN > struct MultiSubjectConfig : afp::DefaultConfig { enum { multiple_subjects = true }; typedef SNN SubjectType; };
DefragStatistics
If defragmentation is configured to collect statistics (defrag_statistics = true
) you can define an individual statistics collection policy DefragStatistics
in each AFP config. It can be useful if you want to get separated statistics for multiple channels.
Example:
struct MyAFPDefragConfig1 : afp::DefaultConfig { enum { // ... defrag_statistics = true }; typedef afp::defrag::Statistics<MyAFPDefragConfig1> DefragStatistics; }; //... // Get current fragment statistics afp::defrag::FragmentStats fs; MyAFPDefagConfig1::DefragStatistics::get_fragment_stats(fs);
Predefined AFP configs
The following table gives an overview on the predefined AFP configuration structs. They are all defined in the namespace famouso::mw::afp
, assuming #include "mw/afp/Config.h"
.
Option | |||
---|---|---|---|
false | true | ||
false | true | ||
false | true | ||
false | |||
false | true | ||
false | |||
true | |||
AVR: 8 | |||
AVR: 8 | |||
AVR: 256 |
Changing AFP configuration used in the Abstract Network Layer
The AFP configuration that is used in the famouso Abstract Network Layer can be changed in the definition of famouso's layer stack. The configurations used for fragmentation and defragmentation can be given in the second and third template parameter of famouso::mw::anl::AbstractNetworkLayer. Both are optional. By default the configuration defined by the Network Layer is used.
Some examples:
- disabling AFP completely:
class config { // ... typedef famouso::mw::nl::CANNL<can, ccpClient, etagClient> nl; typedef famouso::mw::anl::AbstractNetworkLayer< nl, famouso::mw::afp::NoFragmentation, // Fragmentation config: disable famouso::mw::afp::NoDefragmentation // Defragmentation config: disable > anl; public: typedef famouso::mw::el::EventLayer< anl > EL; typedef famouso::mw::api::PublisherEventChannel<EL> PEC; typedef famouso::mw::api::SubscriberEventChannel<EL> SEC; };
- disabling defragmentation only:
class config { // ... typedef famouso::mw::nl::CANNL<can, ccpClient, etagClient> nl; typedef famouso::mw::anl::AbstractNetworkLayer< nl, nl::AFP_Config, // Fragmentation config: default famouso::mw::afp::NoDefragmentation // Defragmentation config: disable > anl; public: typedef famouso::mw::el::EventLayer< anl > EL; typedef famouso::mw::api::PublisherEventChannel<EL> PEC; typedef famouso::mw::api::SubscriberEventChannel<EL> SEC; };
- changing fragmentation and defragmentation config:
class config { // ... typedef famouso::mw::nl::CANNL<can, ccpClient, etagClient> nl; struct afp_cfg : nl::AFP_Config { enum { overflow_error_checking = false }; typedef afp::DefaultEventSizeProp SizeProperties; }; typedef famouso::mw::anl::AbstractNetworkLayer< nl, afp_cfg, // Own fragmentation config afp_cfg // Own defragmentation config > anl; public: typedef famouso::mw::el::EventLayer< anl > EL; typedef famouso::mw::api::PublisherEventChannel<EL> PEC; typedef famouso::mw::api::SubscriberEventChannel<EL> SEC; };
AFP configuration in the Network Layer
Due to the fact that the network type and its characteristics influences the steps to take for ensuring correct reassembly of fragmented events, the default AFP configuration for the Abstract Network Layer is defined by each Network Layer individually.
Writing a Network Layer, you firstly have to #include "mw/afp/Config.h"
. Secondly you have to define the public type AFP_Config
in your NL class. It's recommended to use typedef
with one of the following predefined configs if there is one that meets the network characteristics.
afp::Disable
: fragmentation and defragmentation support is disabled by default.afp::MultiSubjectConfig<SNN>
: use no event sequence numbering, do not support handling of reordered packets, do not check for duplicate fragments. Choose this, if there are neither reordering of packets nor duplicates and the probability of packet loss is very low.afp::MultiSubjectESeqReorderDuplicateConfig<SNN>
: use event sequence numbering, support handling of reordered packets, check for duplicate fragments. Choose this, if there is both reordering and duplicates.
To create an own config see How to configure AFP and Configuration options and use afp::MultiSubjectConfig<SNN>
as base class (multiple_subjects = true
is needed in a default config for the Abstract Network Layer).